This article explores various techniques to handle undefined properties within EJS templates used in ExpressJS applications. It guides you on how to gracefully manage scenarios where data passed to the template might lack specific properties, preventing errors and ensuring a smooth user experience.
We will discuss different types of approaches to check for undefined properties in EJS for ExpressJS.
Table of Content
Using Conditional Statements (if-else):
We can directly use an if statement to check if a variable exists before attempting to access its properties.
- With this approach, we can use traditional if-else statements to check if a property is undefined and then render content based on the condition.
- This method provides flexibility in handling different scenarios by allowing us to specify distinct actions for when a property is defined and when it's undefined
<% if (user.name !== undefined) { %>
<h1>Welcome, <%= user.name %>!</h1>
<% } else { %>
<h1>Welcome, Guest!</h1>
<% } %>
Using Ternary Operators:
The ternary operator provides a concise way to conditionally render content based on the existence of a property.
- Ternary operators offer a concise way to handle undefined properties by providing a conditional expression.
- This approach is particularly useful for simpler conditions and scenarios where you want to keep your code compact.
<h1>Welcome, <%= user.name !== undefined ? user.name : 'Guest' %>!</h1>
Implementing Default Values with the || Operator:
The typeof operator can be employed to check the type of a variable and handle undefined properties accordingly.
- The || (OR) operator allows us to set default values for undefined properties, ensuring a fallback option.
- This approach is straightforward and efficient, providing a clean way to handle missing data.
<h1>Welcome, <%= user.name || 'Guest' %>!</h1>
Using the typeof Operator:
We can use the || (OR) operator to provide a default value in case a property is undefined.
- By using the typeof operator, we can check the type of a variable and handle undefined properties accordingly.
- This approach is particularly useful when we need to handle different data types or perform additional checks based on property existence.
<% if (typeof user.age !== 'undefined') { %>
<p>Your age: <%= user.age %></p>
<% } else { %>
<p>Your age: Not specified</p>
<% } %>
Note:- Each of these approaches offers its own advantages and is suitable for different use cases. It's essential to choose the approach that best fits your requirements in terms of readability, maintainability, and performance.
Steps to Create Express JS App & Installing Module:
Step 1: Create a new directory for your project:
mkdir ejs-undefined-properties
Step 2: Navigate into the project directory:
cd ejs-undefined-properties
Step 3: Initialize npm (Node Package Manager) to create a package.json file:
npm init -y
Step 4: Install required modules (Express and EJS) using npm:
npm install express ejs
Project Structure:
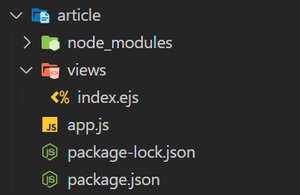
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2"
}
Example: Below is an example of hacking undefined property in EJS for nodeJS.
- Case 1: When both user.name and user.age are defined:
Welcome, GFG!
Your age: 30
- Case 2: When user.name is undefined but user.age is defined:
Welcome, GuestGFG!
Your age: 30
- Case 3: When both user.name and user.age are undefined:
Welcome, Guest!
Your age: Not specified
<!-- views/index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Handling Undefined Properties</title>
</head>
<body>
<h1>Welcome, <%= user.name || 'Guest' %>!</h1>
<p>Your age: <%= user.age ? user.age : 'Not specified' %>
</p>
</body>
</html>
const express = require('express');
const app = express();
const port = 3000;
app.set('view engine', 'ejs');
app.get('/', (req, res) => {
const user = { name: 'GFG'};
res.render('index', { user });
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Output:
.gif)
Output:- when age is undefined