This article focuses on discussing how to add Cascading Style Sheets (CSS) in Ruby on Rails.
Table of Content
Using SCSS
SCSS (Sass) is a preprocessor that extends CSS with features like variables, mixins, and nesting. These features make your stylesheets more maintainable, reusable, and easier to read.
1. Create SCSS File: Start by creating a new file with the .scss extension in the app/assets/stylesheets directory.
2. Import Bootstrap (Optional): If you're using Bootstrap for pre-built styles, import it into your SCSS file using @import "bootstrap";
3. Write SCSS Styles: Add your custom styles to the SCSS file. Utilize features like variables, mixins, and nesting for better organization:
application.scss
body {
font-family: "Arial", sans-serif;
background-color: #b6d3f1;
}
div {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
p {
font-size: 20px;
}
4. Link SCSS File: In your application layout file (usually app/views/layouts/application.html.erb), use the stylesheet_link_tag helper to link the SCSS file:
<%= stylesheet_link_tag 'application', media: 'all', data: { turbolinks: false } %>
Below example shows the content of app/views/layouts/application.html.erb:
<!DOCTYPE html>
<html>
<head>
<title>Rails App with SCSS</title>
<%= stylesheet_link_tag 'application', media: 'all', data: { turbolinks: false } %>
</head>
<body>
<div>
<p>Welcome to Ruby and Rails application</p>
</div>
</body>
</html>
Output:
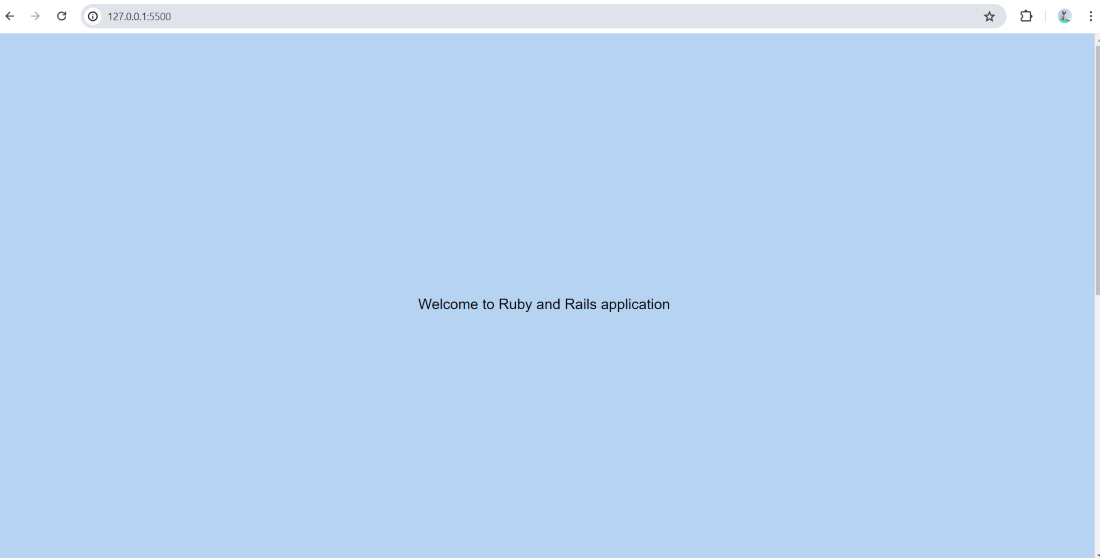
Output
Using Plain CSS
While SCSS is generally preferred for its advanced features, you can also use plain CSS files.
1. Create CSS File: Create a new file with the .css extension in the app/assets/stylesheets directory (e.g., custom.css).
2. Write CSS Styles: Add your custom styles directly to the CSS file:
custom.css
body {
font-family: 'Arial', sans-serif;
background-color: #f8f9fa;
}
div {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.btn-primary {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border-radius: 5px;
}
3. Link CSS File: Link the CSS file in your application layout using the stylesheet_link_tag helper:
<%= stylesheet_link_tag 'custom', media: 'all', data: { turbolinks: false } %>
Below example shows the content of app/views/layouts/application.html.erb:
<!DOCTYPE html>
<html>
<head>
<title>Rails App with Plain CSS</title>
<%= stylesheet_link_tag 'custom', media: 'all', data: { turbolinks: false } %>
</head>
<body>
<button class="btn-primary" >Click Me</button>
</body>
</html>
Output:
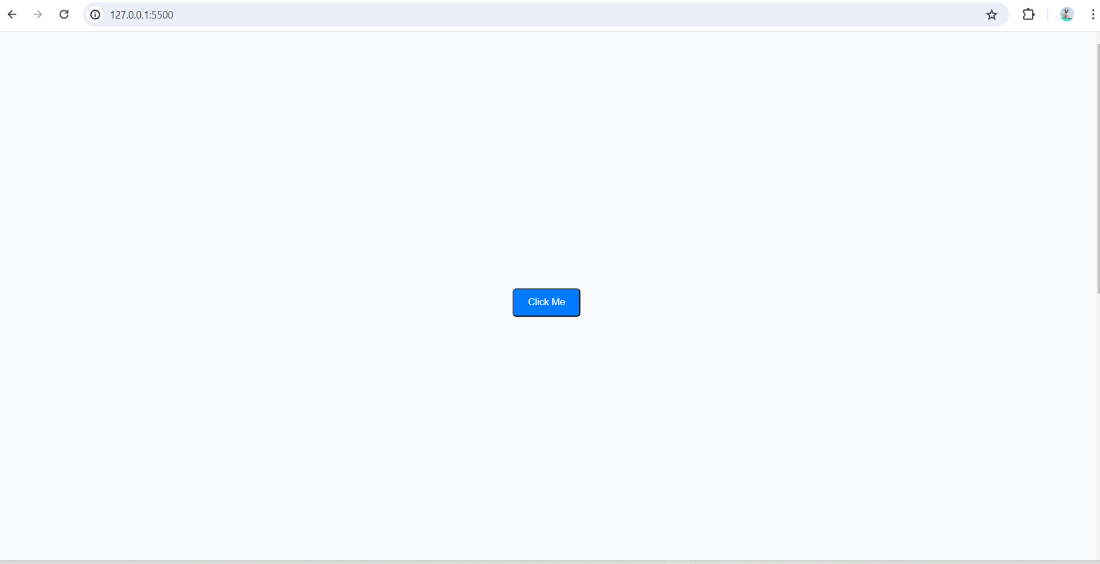
output
Using Inline Styles
Alternatively, you can also apply styles directly within your HTML views using inline styles.
1. Write Inline Styles: Apply styles directly within your HTML views using the style attribute.
Below example shows inline styles in HTML view:
<!DOCTYPE html>
<html>
<head>
<title>Inline Styles Example</title>
</head>
<body style="background-color: #f0f0f0;">
<h1 style="color: blue;">Welcome to my Rails application!</h1>
</body>
</html>
Output:
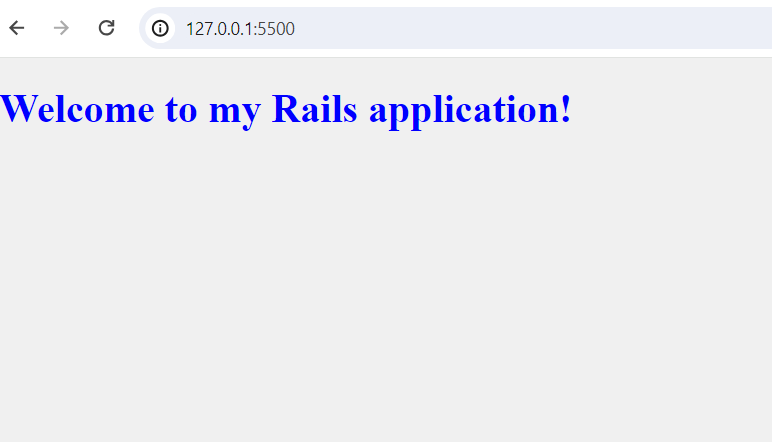
output
Using External CSS Frameworks
Integrating external CSS frameworks like Bootstrap or Foundation can expedite styling and provide a consistent UI across your application.
- Install Framework: Install the desired framework via npm or include its CDN link in your application.
- Follow Framework Guidelines: Refer to the documentation of the chosen framework for instructions on usage and customization.
- Apply Framework Classes: Utilize pre-defined classes provided by the framework to style your elements.
You can install Bootstrap using npm or yarn
npm install bootstrap
Alternatively, you can include the Bootstrap CDN link directly in your application layout
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
Below example shows Bootstrap integration:
<!DOCTYPE html>
<html>
<head>
<title>Rails App with Bootstrap</title>
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container d-flex justify-content-center align-items-center min-vh-100">
<button class="btn-success btn-lg">Welcome to GFG</button>
</div>
</body>
</html>
Output:
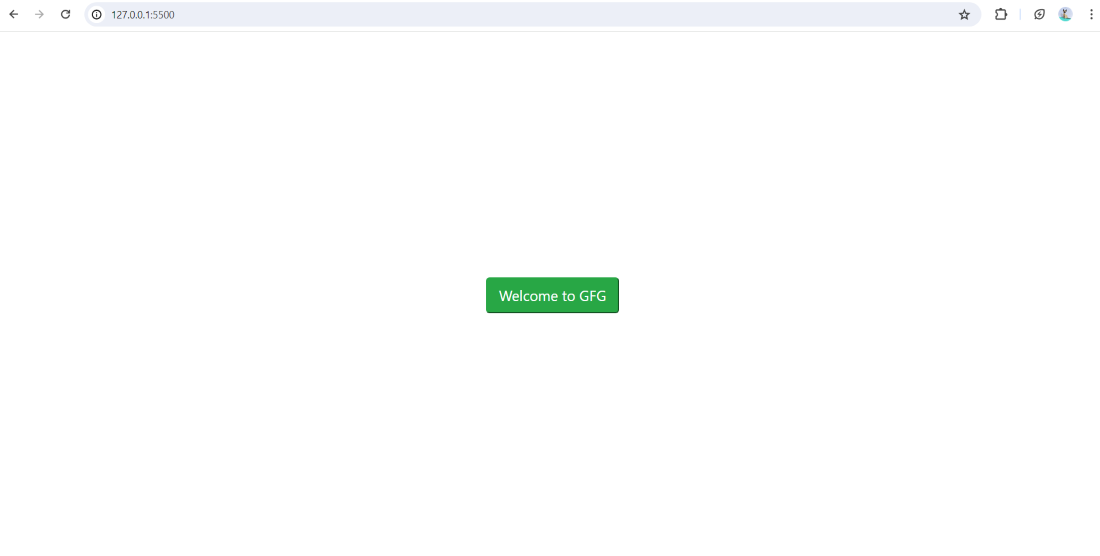
Output
Comparison of Different Approaches
Feature | SCSS | Plain CSS | Inline Styles | External CSS Frameworks |
---|---|---|---|---|
Variables | Yes (Improve maintainability) | No | No | Yes (Framework-specific) |
Mixins | Yes (Reusable code blocks) | No | No | No |
Nesting | Yes (Organize styles hierarchically) | No | No | No |
Preprocessing | Requires compilation step (Rails Asset Pipeline) | No compilation needed | No compilation needed | No compilation needed |
Learning Curve | Slightly steeper learning curve | Easier to learn for beginners | Easy to learn for beginners | Varies based on framework |
Additional Considerations
- Asset Pipeline: Rails uses the Asset Pipeline to manage and compile SCSS/CSS files. The Asset Pipeline concatenates and minifies CSS files for production, improving performance.
- Turbolinks: Use data: { turbolinks: false } in the stylesheet_link_tag to prevent Turbolinks from interfering with CSS reloading during development.
Conclusion
By considering the differences between SCSS, plain CSS, inline styles, and external CSS frameworks, along with the specific needs of your project, you can make an informed decision on which method to use for styling your Ruby on Rails application.