In Angular, *ngFor and @for are constructs used for iterating over collections and rendering dynamic content, but they differ in their implementation and usage. *ngFor is a structural directive that has been a longstanding feature, while @for is a new shorthand syntax introduced in Angular 17. *ngFor is a more verbose and explicit approach, akin to a tried-and-true tool in your toolbox, whereas @for provides a more concise and streamlined way of achieving the same functionality, resembling a sleek, modern gadget that simplifies the process of rendering dynamic lists or repeating elements.
Steps to Create Angular Application
To implement both approaches follow the below steps
Step 1: Create a new angular application
ng new my-app
Folder Structure:
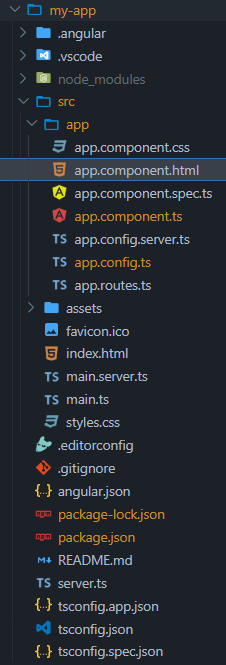
folder structure
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Open app.component.ts and paste the following data which we will use later on during the implementation.
const users = [
{ name: 'John Doe', age: 32 },
{ name: 'Jane Smith', age: 28 },
{ name: 'Bob Johnson', age: 45 }
];
*ngFor
*ngFor is a structural directive in Angular that facilitates the rendering of a collection of items in the template by iterating over an array or an iterable object. It provides a declarative approach to dynamically generate a set of elements based on the elements in the collection. The syntax involves using the *ngFor directive along with a microsyntax that specifies the iteration variable and the source collection. This directive is a built-in feature in Angular and must be imported from the CommonModule or included in the imports array of the respective module or standalone component before it can be utilized in the template.
Syntax:
<element *ngFor="let item of items; let i=index">
<!-- content -->
</element>
Features of *ngFor:
- Supports iterating over arrays, objects, and other iterables.
- Provides access to the current item and its index.
- Allows you to use local variables for the item and index.
- Supports additional properties like trackBy for change detection optimization.
Code Example: Open app.component.html and insert below code
<!-- app.component.html -->
<ul>
<li *ngFor="let user of users; let i=index">
{{ i + 1 }}. {{ user.name }}
</li>
</ul>
Output:
@for
@for is a new directive introduced in Angular 17 that provides a concise alternative to the traditional *ngFor structural directive for rendering collections in templates. It offers a streamlined approach to iterating over arrays or iterables. This feature aims to enhance the productivity by allowing for more concise template expressions when rendering dynamic content based on collections, ultimately contributing to more readable and maintainable code.
Syntax:
@for (item of items; track $index) {
<element>
{{ item }}
</element>
}
Features of @for:
- Shorter syntax compared to *ngFor.
- Supports iterating over arrays and iterables.
- Does not provide access to the index by default (you can still use the index property if needed).
- No additional properties like trackBy.
Code Example: Open app.component.html and insert below code
<!-- app.component.html -->
<ul>
@for (user of users; track $index) {
<li>
{{ user.name }}
</li>
}
</ul>
Output:
Differences between *ngFor and @for
Feature | *ngFor | @for |
---|---|---|
Syntax | Longer syntax with *ngFor | Shorter syntax with @for |
Iterable Types | Arrays, objects, and other iterables | Arrays and iterables |
Index Access | Provides index access by default | No index access by default |
Additional Properties | Supports trackBy and other properties | No additional properties |
Use Case | Suitable for complex templates and scenarios | Suitable for simple templates and scenarios |
Summary
Both ngFor and @for are used to repeat elements in Angular templates based on a list. However, they have some differences in how they work and when to use them. ngFor has a longer way of writing it, but it provides more options like accessing the index of each item in the list and optimizing how Angular keeps track of changes. ngFor is a good choice when you have more complex templates. On the other hand, @for has a shorter way of writing it, making it easier to read. But it doesn't have as many extra options as ngFor. @for is a good choice when you have simpler templates and don't need those extra options.