Both for
loops and while
loops are control flow structures in programming that allow you to repeatedly execute a block of code. However, they differ in their syntax and use cases. It is important for a beginner to know the key differences between both of them.
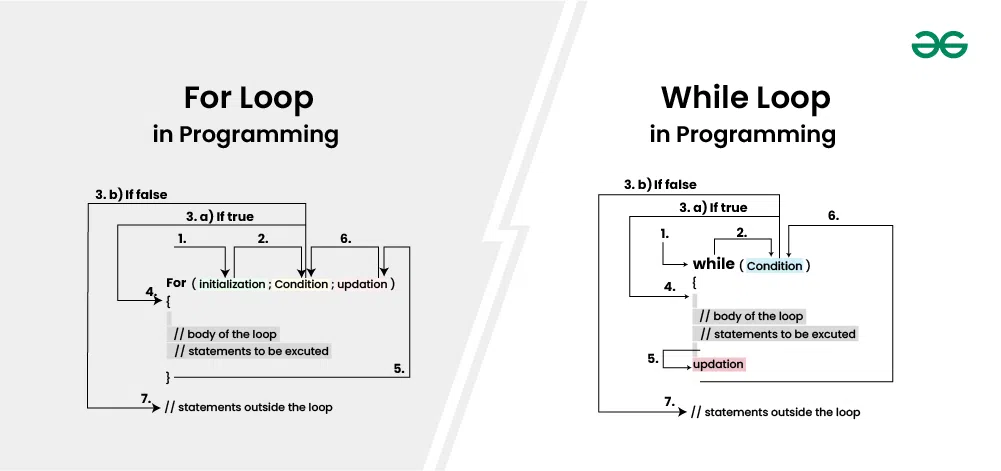
Difference between For Loop and While Loop
For Loop in Programming:
- The
for
loop is used when you know in advance how many times you want to execute the block of code. - It iterates over a sequence (e.g., a list, tuple, string, or range) and executes the block of code for each item in the sequence.
- The loop variable (
variable
) takes the value of each item in the sequence during each iteration.
Example:
#include <iostream>
using namespace std;
int main()
{
for (int i = 0; i < 5; i++)
cout << i << "\n";
return 0;
}
#include <stdio.h>
int main()
{
for (int i = 0; i < 5; i++) {
printf("%d\n", i);
}
return 0;
}
public class Main {
public static void main(String[] args) {
// For loop to print numbers from 0 to 4
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
}
}
for i in range(5):
print(i)
// For loop to print numbers from 0 to 4
for (let i = 0; i < 5; i++) {
console.log(i);
}
Output
0 1 2 3 4
This prints the numbers 0 through 4.
While Loop in Programming:
- The
while
loop is used when you don't know in advance how many times you want to execute the block of code. It continues to execute as long as the specified condition is true. - It's important to make sure that the condition eventually becomes false; otherwise, the loop will run indefinitely, resulting in an infinite loop.
Example:
#include <iostream>
using namespace std;
int main()
{
int count = 0;
while (count < 5) {
cout << count << "\n";
count += 1;
}
cout << endl;
return 0;
}
#include <stdio.h>
int main()
{
int count = 0;
while (count < 5) {
printf("%d\n", count);
count += 1;
}
return 0;
}
public class Main {
public static void main(String[] args) {
int count = 0;
// Example: While loop to print numbers from 0 to 4
while (count < 5) {
System.out.println(count);
count += 1;
}
System.out.println();
}
}
count = 0
while count < 5:
print(count)
count += 1
let count = 0;
while (count < 5) {
console.log(count);
count += 1;
}
console.log();
Output
0 1 2 3 4
This prints the numbers 0 through 4, similar to the for
loop example.
Difference between For Loop and While Loop in Programming:
Key differences between for
and while
loops:
Feature | for Loop | while Loop |
---|---|---|
Initialization | Declared within the loop structure and executed once at the beginning. | Declared outside the loop; should be done explicitly before the loop. |
Condition | Checked before each iteration. | Checked before each iteration. |
Update | Executed after each iteration. | Executed inside the loop; needs to be handled explicitly. |
Use Cases | Suitable for a known number of iterations or when looping over ranges. | Useful when the number of iterations is not known in advance or based on a condition. |
Initialization and Update Scope | Limited to the loop body. | Scope extends beyond the loop; needs to be handled explicitly. |
Choose between for
and while
loops based on the specific requirements of your program and the nature of the problem you are solving.