In Angular, asynchronous programming plays a crucial role in handling data streams and managing side effects. Two common approaches for handling asynchronous data with observables are using the async operator in templates and subscribing to observables in component classes. In this article, we'll see more about the differences between these two approaches, exploring their syntax, features, and use cases.
Table of Content
Steps to Create Angular Application
To implement both of them, follow the below steps
Step 1: Create a new angular application
ng new my-app
Folder Structure:
Dependencies
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/platform-server": "^17.2.0",
"@angular/router": "^17.2.0",
"@angular/ssr": "^17.2.3",
"express": "^4.18.2",
"ngx-webstorage": "^13.0.1",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Async Operator
The async operator is a pipe in Angular that automatically subscribes to an Observable and unsubscribes when the component is destroyed. It is a convenient way to handle Observables in the component's template.
Syntax
{{ observable$ | async }}
Features of Async Operator
- Automatic subscription and unsubscription management.
- Seamless integration with Angular's change detection mechanism.
- Simplifies asynchronous data handling in templates, reducing component logic.
- Prevents memory leaks by automatically unsubscribing from observables.
- Enhances code readability and maintainability by encapsulating asynchronous behavior in templates.
Example
<!-- app.component.html -->
<p>Current Value: {{ value$ | async }}</p>
// app.component.ts
import { Component } from '@angular/core';
import { Observable, interval } from 'rxjs';
import { AsyncPipe, NgFor } from '@angular/common';
@Component({
selector: 'app-root',
standalone: true,
imports: [NgFor, AsyncPipe],
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
value$: Observable<number> = interval(1000);
}
Output:
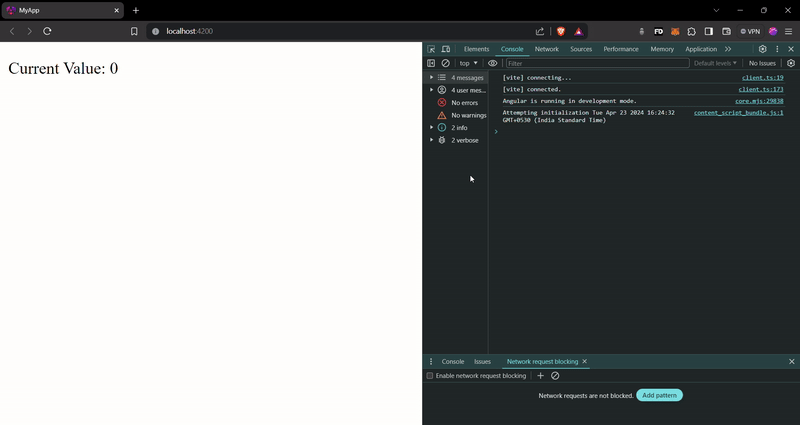
Subscribing to Observable
Subscribing to an Observable involves creating a subscription that listens for emissions from the Observable and executes the provided callback functions.
Syntax
observable.subscribe(
nextValueCallback,
errorCallback,
completeCallback
);
Features of Subscribing to Observable
- Provides core control over asynchronous data handling and side effects.
- Allows for error handling and completion logic.
- Enables the execution of additional code upon emission of values.
- Manual subscription management, requiring explicit unsubscription to prevent memory leaks.
- Suitable for scenarios where complex data manipulation or side effects are required.
Example
// app.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { Observable, interval, Subscription } from 'rxjs';
@Component({
standalone: true,
imports: [],
selector: 'app-example',
template: '<p>Current Value: {{ currentValue }}</p>'
})
export class AppComponent implements OnInit, OnDestroy {
currentValue: number = 0;
subscription!: Subscription;
ngOnInit() {
const value$: Observable<number> = interval(1000).pipe(take(10));
this.subscription = value$.subscribe(
(value) => this.currentValue = value,
(error) => console.error(error),
() => console.log('Observable completed')
);
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
}
Output:
Difference between async operator and subscribing to observable
Aspect | Async Operator | Subscribing to Observable |
---|---|---|
Subscription Management | Automatic subscription and unsubscription | Manual subscription and unsubscription |
Usage | In component templates | In component code |
Callback Functions | Not provided | Provided for next value, error, and complete events |
Change Detection | Automatic | Manual updates required |
Control | Less control over subscription lifecycle | More control over subscription lifecycle |
Memory Management | Automatically unsubscribes, preventing memory leaks | Requires manual unsubscription to prevent memory leaks |
Conclusion
The async operator and subscribing to an Observable are two different approaches to working with Observables in Angular. The async operator is a convenient way to handle Observables in the component's template, automatically subscribing and unsubscribing. In contrast, subscribing to an Observable directly provides more control over the subscription lifecycle and allows for custom handling of emissions, errors, and completion events.
The choice between the two approaches depends on the specific requirements of the application and the developer's preferences. The async operator is generally preferred for simple use cases in the template, while subscribing to an Observable directly is more suitable for complex scenarios or when more control is required over the subscription lifecycle.