Dark Mode for websites enhances user experience by providing a visually comfortable environment, especially in low-light conditions. Implemented with HTML, Bootstrap, and JavaScript, it offers a sleek interface that reduces eye strain and promotes readability, offering users greater accessibility.
Prerequisites
Approach
- Here we are using Bootstrap 5.1.3 and Bootstrap 4.5.2 for styling and layout.
- Two <div> elements with different background colors contain content about Bootstrap and JavaScript.
- Toggles between dark and light modes using JavaScript functions.
- Utilizes Bootstrap buttons with btn-primary and btn-secondary classes.
- Ensures responsiveness across different screen sizes with Bootstrap's grid system.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>
Creating Dark Mode for Websites
using HTML Bootstrap & JavaScript ?
</title>
<!-- Bootstrap CSS -->
<link href=
"https://stackpath.bootstrapcdn.com/bootstrap/5.1.3/css/bootstrap.min.css"
rel="stylesheet">
<!-- Bootstrap 4 CSS (this should be removed if not needed) -->
<link href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body class="light-mode" style="background-color: white;">
<div class="container">
<h1 class="mt-5">GeeksForGeeks</h1>
<p>
This is a sample GeeksForGeeks Text.
Dark Mode Websites using Html & Bootstrap and JS
</p>
<div class="row mb-3 mb-md-0">
<div class="col-md-6 mb-3 mb-md-0">
<div class="p-3 text-white" style="background-color: #007bff;">
<h2>Bootstrap</h2>
<p>
Bootstrap is a free and open-source tool
collection for creating responsive websites
and web applications. It is the most popular
HTML, CSS, and JavaScript framework for
developing responsive, mobile-first websites.
It solves many problems which we had once,
one of which is the cross-browser compatibility
issue. Nowadays, the websites are perfect for
all the browsers (IE, Firefox, and Chrome) and
for all sizes of screens (Desktop, Tablets,
Phablets, and Phones).
</p>
</div>
</div>
<div class="col-md-6 mb-3 mb-md-0">
<div class="p-3 text-white"
style="background-color: #28a745;">
<h2>JavaScript</h2>
<p>
JavaScript is a lightweight, cross-platform,
single-threaded, and interpreted compiled
programming language. It is also known as the
scripting language for webpages. It is well-known
for the development of web pages, and many non-browser
environments also use it.
JavaScript is a weakly typed language (dynamically typed).
JavaScript can be used for Client-side developments
as well as Server-side developments.
</p>
</div>
</div>
</div>
<h3 id="DarkModetext">Dark Mode is OFF</h3>
<!-- Buttons for toggling light and
dark modes with enhanced Bootstrap classes -->
<div class="d-flex justify-content-center mb-3">
<button class="btn btn-primary btn-lg me-2 mx-2 mb-2"
onclick="toggleDarkMode()">
Dark Mode
</button>
<button class="btn btn-secondary btn-lg mx-2 mb-2"
onclick="toggleLightMode()">
Light Mode
</button>
</div>
</div>
<!-- Bootstrap Bundle with Popper -->
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.1.3/js/bootstrap.bundle.min.js">
</script>
<script>
function toggleDarkMode() {
let element = document.body;
let content = document.getElementById("DarkModetext");
// Switch to dark mode
element.classList.remove("light-mode");
element.classList.add("dark-mode");
element.style.backgroundColor = "black";
document.querySelectorAll('h1, h3, p')
.forEach(el => el.style.color = 'white');
content.innerText = "Dark Mode is ON";
}
function toggleLightMode() {
let element = document.body;
let content = document.getElementById("DarkModetext");
// Switch to light mode
element.classList.remove("dark-mode");
element.classList.add("light-mode");
element.style.backgroundColor = "white";
document.querySelectorAll('h1, h3, p')
.forEach(el => el.style.color = 'black');
content.innerText = "Dark Mode is OFF";
}
</script>
</body>
</html>
Output:
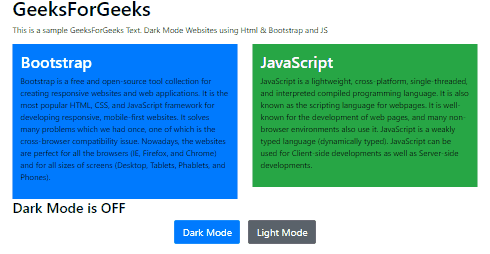
Dark Mode for Websites Example Output