Bitwise algorithms refer to the use of bitwise operators to manipulate individual bits of data. Python provides a set of bitwise operators such as AND (&), OR (|), XOR (^), NOT (~), shift left (<<), and shift right (>>). These operators are commonly used in tasks like encryption, compression, graphics, communications over ports and sockets, embedded systems programming, and more. They can lead to more efficient code when used appropriately.
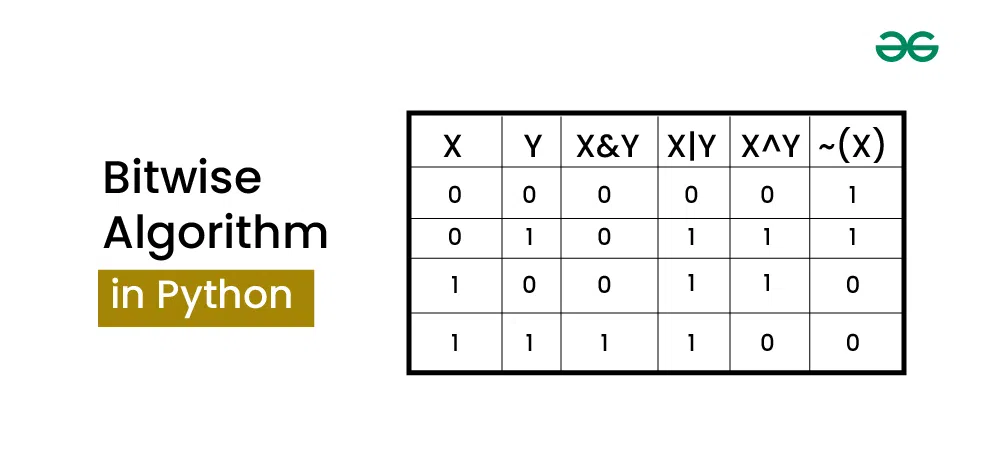
Bitwise Algorithm in Python
What is Bitwise Algorithm?
A bitwise algorithm is a type of algorithm that operates on individual bits of data rather than on larger data types like integers or floating-point numbers. These algorithms manipulate bits directly, typically using bitwise operators such as AND, OR, XOR, left shift, right shift, and complement.
Common Bitwise Operator:
1. Bitwise AND (&
) Operator:
The bitwise AND operator is denoted using a single ampersand symbol, i.e. &. The & operator takes two equal-length bit patterns as parameters. The two-bit integers are compared. If the bits in the compared positions of the bit patterns are 1, then the resulting bit is 1. If not, it is 0.
Implementation of AND operator:
a = 7
b = 4
result = a & b
print(result)
# This code is contributed by akashish__
Output
4
2. Bitwise OR (|
) Operator:
The | Operator takes two equivalent length bit designs as boundaries; if the two bits in the looked-at position are 0, the next bit is zero. If not, it is 1.
Implementation of OR operator:
a = 12
b = 25
result = a | b
print(result)
# This code is contributed by garg28harsh.
Output
29
3. Bitwise XOR (^
) Operator:
The ^ operator (also known as the XOR operator) stands for Exclusive Or. Here, if bits in the compared position do not match their resulting bit is 1. i.e, The result of the bitwise XOR operator is 1 if the corresponding bits of two operands are opposite, otherwise 0.
Implementation of XOR operator:
a = 12
b = 25
result = a ^ b
print(result)
# This code is contributed by garg28harsh.
Output
21
4. Bitwise NOT (~
) Operator:
All the above three bitwise operators are binary operators (i.e, requiring two operands in order to operate). Unlike other bitwise operators, this one requires only one operand to operate.
The bitwise Not Operator takes a single value and returns its one’s complement. The one’s complement of a binary number is obtained by toggling all bits in it, i.e, transforming the 0 bit to 1 and the 1 bit to 0.
Implementation of NOT operator:
a = 0
print("Value of a without using NOT operator: " , a)
print("Inverting using NOT operator (with sign bit): " , (~a))
print("Inverting using NOT operator (without sign bit): " , int(not(a)))
# This code is contributed by akashish__
Output
Value of a without using NOT operator: 0 Inverting using NOT operator (with sign bit): -1 Inverting using NOT operator (without sign bit): 1
5. Left-Shift (<<) Operator:
The left shift operator is denoted by the double left arrow key (<<). The general syntax for left shift is shift-expression << k. The left-shift operator causes the bits in shift expression to be shifted to the left by the number of positions specified by k. The bit positions that the shift operation has vacated are zero-filled.
Implementation of Left shift operator:
# Python code for the above approach
num1 = 1024
bt1 = bin(num1)[2:].zfill(32)
print(bt1)
num2 = num1 << 1
bt2 = bin(num2)[2:].zfill(32)
print(bt2)
num3 = num1 << 2
bitset13 = bin(num3)[2:].zfill(16)
print(bitset13)
# This code is contributed by Prince Kumar
Output
00000000000000000000010000000000 00000000000000000000100000000000 0001000000000000
6. Right-Shift (>>) Operator:
The right shift operator is denoted by the double right arrow key (>>). The general syntax for the right shift is “shift-expression >> k”. The right-shift operator causes the bits in shift expression to be shifted to the right by the number of positions specified by k. For unsigned numbers, the bit positions that the shift operation has vacated are zero-filled. For signed numbers, the sign bit is used to fill the vacated bit positions. In other words, if the number is positive, 0 is used, and if the number is negative, 1 is used.
Implementation of Right shift operator:
def main():
num1 = 1024
bt1 = bin(num1)[2:].zfill(32)
print(bt1)
num2 = num1 >> 1
bt2 = bin(num2)[2:].zfill(32)
print(bt2)
num3 = num1 >> 2
bitset13 = bin(num3)[2:].zfill(16)
print(bitset13)
if __name__ == "__main__":
main()
Output
00000000000000000000010000000000 00000000000000000000001000000000 0000000100000000
Bit Manipulation Techniques:
1. Checking if a Bit is Set:
To check if a specific bit is set in an integer:
Implementation of Checking if a Bit is Set:
def is_bit_set(num, pos):
return (num & (1 << pos)) != 0
num = 5 # 0101 in binary
print(is_bit_set(num, 0)) # Check if the least significant bit is set
# Output: True
print(is_bit_set(num, 1)) # Check if the second least significant bit is set
# Output: False
Output
True False
2. Setting a Bit:
To set a specific bit in an integer:
Implementation of setting a bit:
def set_bit(num, pos):
return num | (1 << pos)
num = 5 # 0101 in binary
result = set_bit(num, 1) # Set the second least significant bit
print(result) # Output: 7 (0111 in binary)
Output
7
3. Clearing a Bit:
Implementation of clearing a bit:
def clear_bit(num, pos):
return num & ~(1 << pos)
num = 7 # 0111 in binary
result = clear_bit(num, 1) # Clear the second least significant bit
print(result) # Output: 5 (0101 in binary)
Output
5
4. Toggling a Bit:
To toggle a specific bit in an integer:
Implementation of toggling a bit:
def toggle_bit(num, pos):
return num ^ (1 << pos)
num = 5 # 0101 in binary
result = toggle_bit(num, 1) # Toggle the second least significant bit
print(result) # Output: 7 (0111 in binary)
Output
7
5. Counting Set Bits:
To count the number of set bits (1s) in an integer:
Implementation of counting set bits:
def count_set_bits(num):
count = 0
while num:
count += num & 1
num >>= 1
return count
num = 7 # 0111 in binary
print(count_set_bits(num)) # Output: 3
Output
3
Related article:
- Bits manipulation (Important tactics)
- Bitwise Hacks for Competitive Programming
- Bit Tricks for Competitive Programming